activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="5dp"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20dp"
android:text="이름 :"/>
<EditText
android:id="@+id/edtName"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"/>
</LinearLayout>
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20dp"
android:text="인원 :"/>
<EditText
android:id="@+id/edtNumber"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
>
<Button
android:id="@+id/btnInit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginRight="5dp"
android:backgroundTint="#50000000"
android:textColor="@color/black"
android:text="초기화"/>
<Button
android:id="@+id/btnInsert"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginRight="5dp"
android:backgroundTint="#50000000"
android:textColor="@color/black"
android:text="입력"/>
<Button
android:id="@+id/btnSeclect"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:backgroundTint="#50000000"
android:textColor="@color/black"
android:text="조회"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
android:gravity="center">
<EditText
android:id="@+id/edtNameResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginRight="25dp"/>
<EditText
android:id="@+id/edtNumberResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
</LinearLayout>
MainActivity.java
package com.example.project12_2;
import androidx.appcompat.app.AppCompatActivity;
import android.app.Activity;
import android.content.Context;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class MainActivity extends Activity {
myDBHelper myHelper;
EditText edtName, edtNumber, edtNameResult, edtNumberResult;
Button btnInit, btnInsert, btnSelect;
SQLiteDatabase sqlDB;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
setTitle("가수 그룹 관리 DB");
edtName = (EditText) findViewById(R.id.edtName);
edtNumber = (EditText) findViewById(R.id.edtNumber);
edtNameResult = (EditText) findViewById(R.id.edtNameResult);
edtNumberResult = (EditText) findViewById(R.id.edtNumberResult);
btnInit = (Button) findViewById(R.id.btnInit);
btnInsert = (Button) findViewById(R.id.btnInsert);
btnSelect = (Button) findViewById(R.id.btnSeclect);
myHelper = new myDBHelper(this);
btnInit.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
sqlDB = myHelper.getWritableDatabase();
//onUpgrade 호출, groupTBL이 있다면 삭제한 후 새로 생성한다
myHelper.onUpgrade(sqlDB,1,2);
//Database를 닫는다
sqlDB.close();
}
});
btnInsert.setOnClickListener(new View.OnClickListener(){
@Override
public void onClick(View view){
//groupDB를 쓰기용으로 연다
sqlDB=myHelper.getWritableDatabase();
sqlDB.execSQL("INSERT INTO groupTBL VALUES ('"+edtName.getText().toString()+"',"+edtNumber.getText().toString()+");");
sqlDB.close();
Toast.makeText(getApplicationContext(),"입력됨",Toast.LENGTH_SHORT).show();
edtName.setText("");
edtNumber.setText("");
}
});
btnSelect.setOnClickListener(new View.OnClickListener(){
public void onClick(View v){
sqlDB = myHelper.getReadableDatabase();
Cursor cursor;
cursor = sqlDB.rawQuery("SELECT * FROM groupTBL;",null);
String strNames = "그룹 이름"+"\r\n"+"--------"+"\r\n";
String strNumbers = "인원"+"\r\n"+"--------"+"\r\n";
while(cursor.moveToNext()){
strNames+=cursor.getString(0)+"\r\n";
strNumbers+=cursor.getString(1)+"\r\n";
}
edtNameResult.setText(strNames);
edtNumberResult.setText(strNumbers);
cursor.close();
sqlDB.close();
}
});
}
public class myDBHelper extends SQLiteOpenHelper{
public myDBHelper(Context context){
// 두번째 파라미터에는 새로 생성될 데이터베이스의 파일명을 지정하면 된다.
super(context, "groupDB",null,1);
}
@Override
// onUpgrade()에서 호출되거나 데이터를 입력할 때 혹은 테이블이 없을 때 처음 1회 호출한다.
public void onCreate(SQLiteDatabase db){
db.execSQL("CREATE TABLE groupTBL ( gName CHAR(20) PRIMARY KEY, gNumber INTEGER);");
}
@Override
//테이블을 삭제하고 새로 생성한다. 초기화할 때 호출한다.
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion){
db.execSQL("DROP TABLE IF EXISTS groupTBL");
onCreate(db);
}
}
}
배운 점
자바 파일에서 SQLiteOpenHelper를 이용하여 직접 DB를 만들 수 있다.
실행 영상
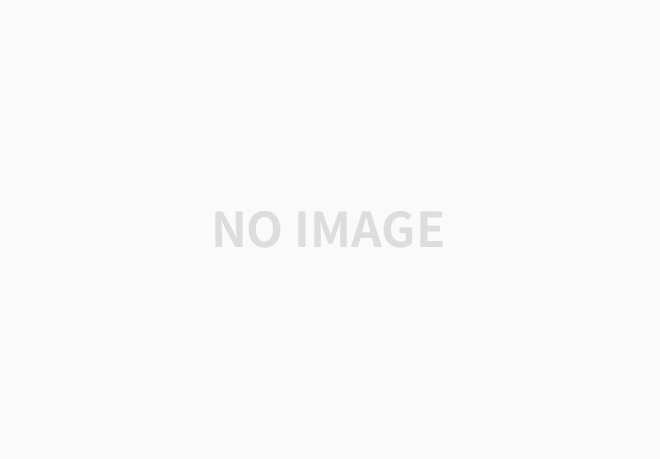
'안드로이드 > 연습' 카테고리의 다른 글
[Android] ProgressBar&SeekBar (0) | 2021.08.29 |
---|---|
[Android] 간단 MP3 플레이어 만들기 (0) | 2021.08.29 |
[Android] 영화 포스터 보기(GridLayout) (0) | 2021.08.27 |
[Android] ListView (0) | 2021.08.27 |
[Android] 새로운 액티비티 추가하기 (0) | 2021.08.26 |